Laravel API Create and test an API for Laravel -(r) (r)
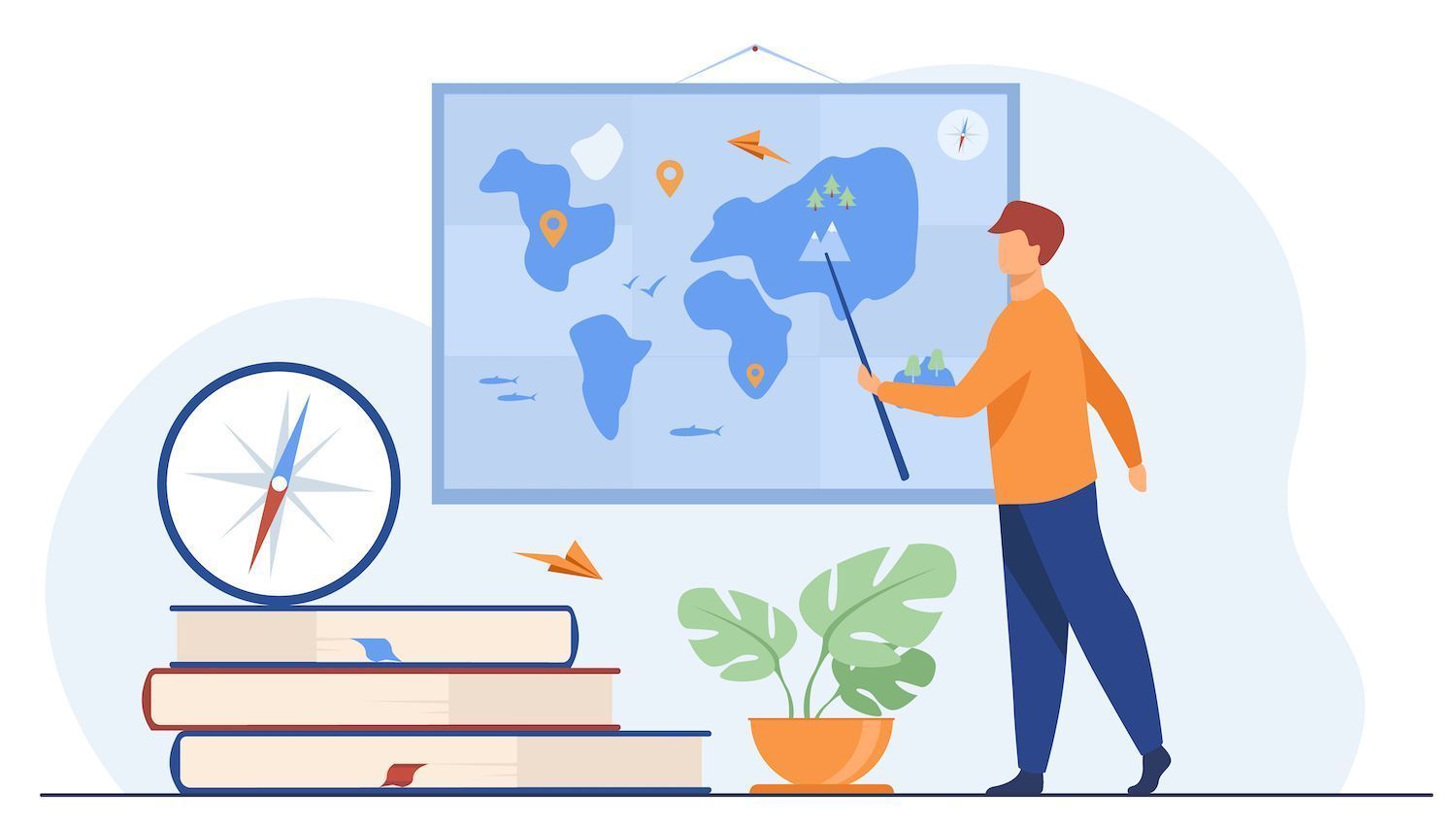
Please forward the news to
Laravel Eloquent can be a straightforward method of connecting to your database. It's an object-relational mapping (ORM) that simplifies the complexity of databases through making a framework that allows you to interact with tables.
So, Laravel Eloquent has excellent tools to create and test APIs. The tools will help you in creating APIs. In this article, you'll learn how simple to develop APIs and test them in Laravel.
The conditions
For you to begin here's what you'll need:
API Basics
Create a brand new Laravel project with the composer
:
composer create-project laravel/laravel laravel-api-create-test
To start the server, execute this command. This starts the server at port 800:
cd laravel-api-create-test php artisan serve
It is highly recommended that you go through the following screen:
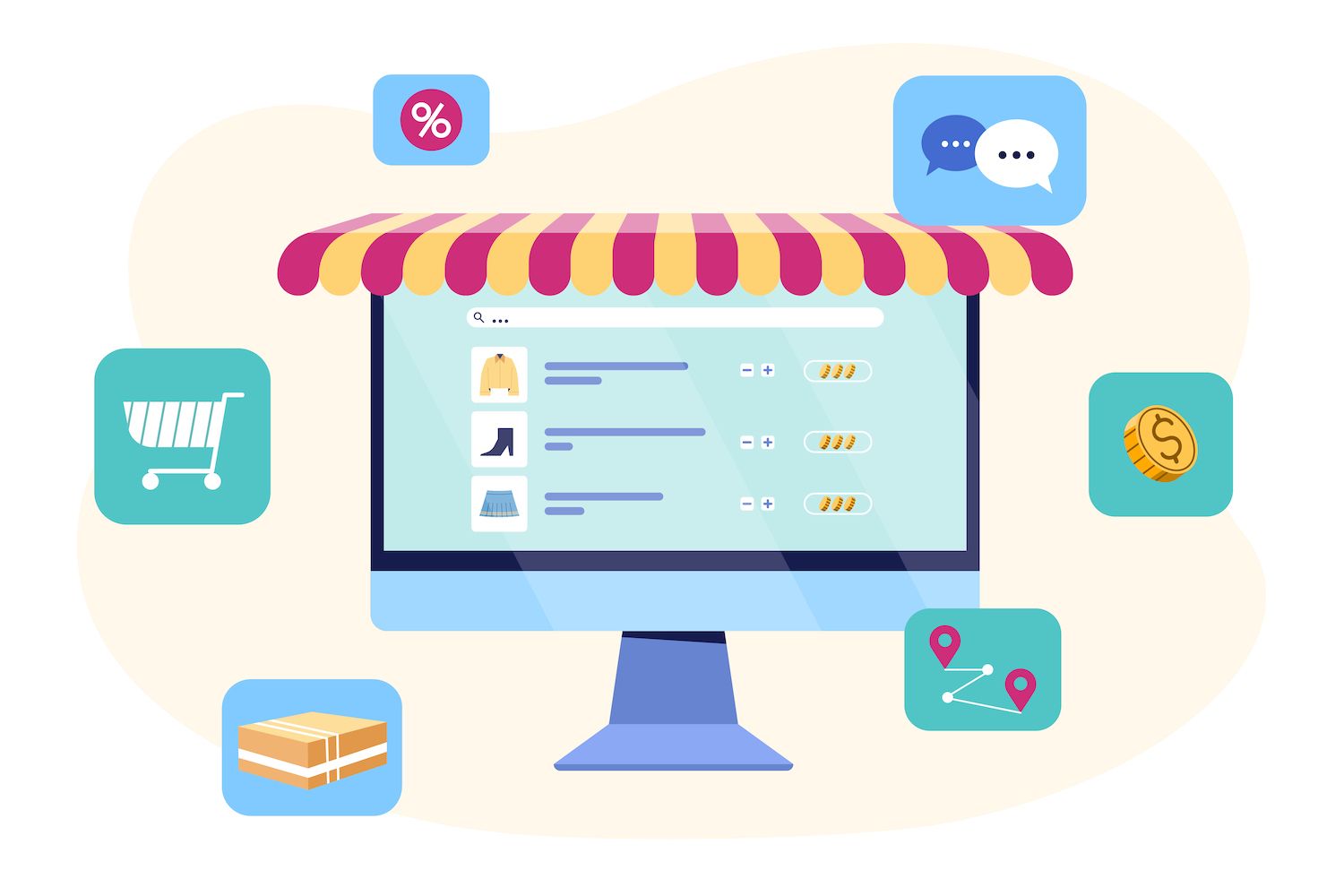
Make models that have the "-m
flag to enable the migration by with the code that is listed as follows:
php artisan make:model Product -m
Then, upgrade your file so that it contains the mandatory field. Add title and description fields for the product model and these two table fields inside the database/migrations/date_stamp_create_products_table.php file.
$table->string('title'); $table->longText('description');
The next step is to make those fields filled to the max. Inside app/Models/Product.php, make title
and description
fillable fields.
protected $fillable = ['title', 'description'];
What do you need to know about the Controller?
Create a controller that can control the device using the command. This will create the app/Http/Controllers/Api/ProductController.php file.
php artisan make:controller Api\\ProductController --model=Product
Develop the logic to create and retrieving objects. Within an index index
method, you could add this code to look at every object.
products = $products + all :(); Return the result ()->json(["status" is"false" and "products" exceeds"$products");
Then, you need to add an StoreProductRequest
class to store the latest items within your database. The following class is to be put on top of the existing file.
public function store(StoreProductRequest $request) $product = Product::create($request->all()); return response()->json([ 'status' => true, 'message' => "Product Created successfully! ", 'product' => $product ], 200);
Following procedure is to begin the process of making the request. This can be done by running this command:
php artisan make:request StoreProductRequest
If you want to add validations, you can use the app/Http/Requests/StoreProductRequest.php file. It's a test that doesn't include validation.
What Are You Required To Design To A Route
The final stage before attempting with an API is to design the new route. In order to do this, include this code into your routes/api.php file. In the file, include the use
declaration at the top of the file. Also, include the Route
assertion in the body
use App\Http\Controllers\Api\ProductController; Route::apiResource('products', ProductController::class);
Before you begin testing the API, be sure there is a products table exists within your database. If it is not there, create this table with the aid of a control panel such like XAMPP. Also, you can use the following commands to transfer the table
php artisan migrate
What's the most efficient way to test an API? API
Before testing the API, ensure that the authorize
method inside the app/Http/Requests/StoreProductRequest.php is set to return true
.
Create a new product with Postman. Start by hitting a POST
request to this URL: http://127.0.0.1:8000/api/products/. As this is an POST request for an
application to facilitate the creation of a new product it is essential to supply an JSON object that includes the name and description.
"title":"Apple", "description":"Best Apples of the world"
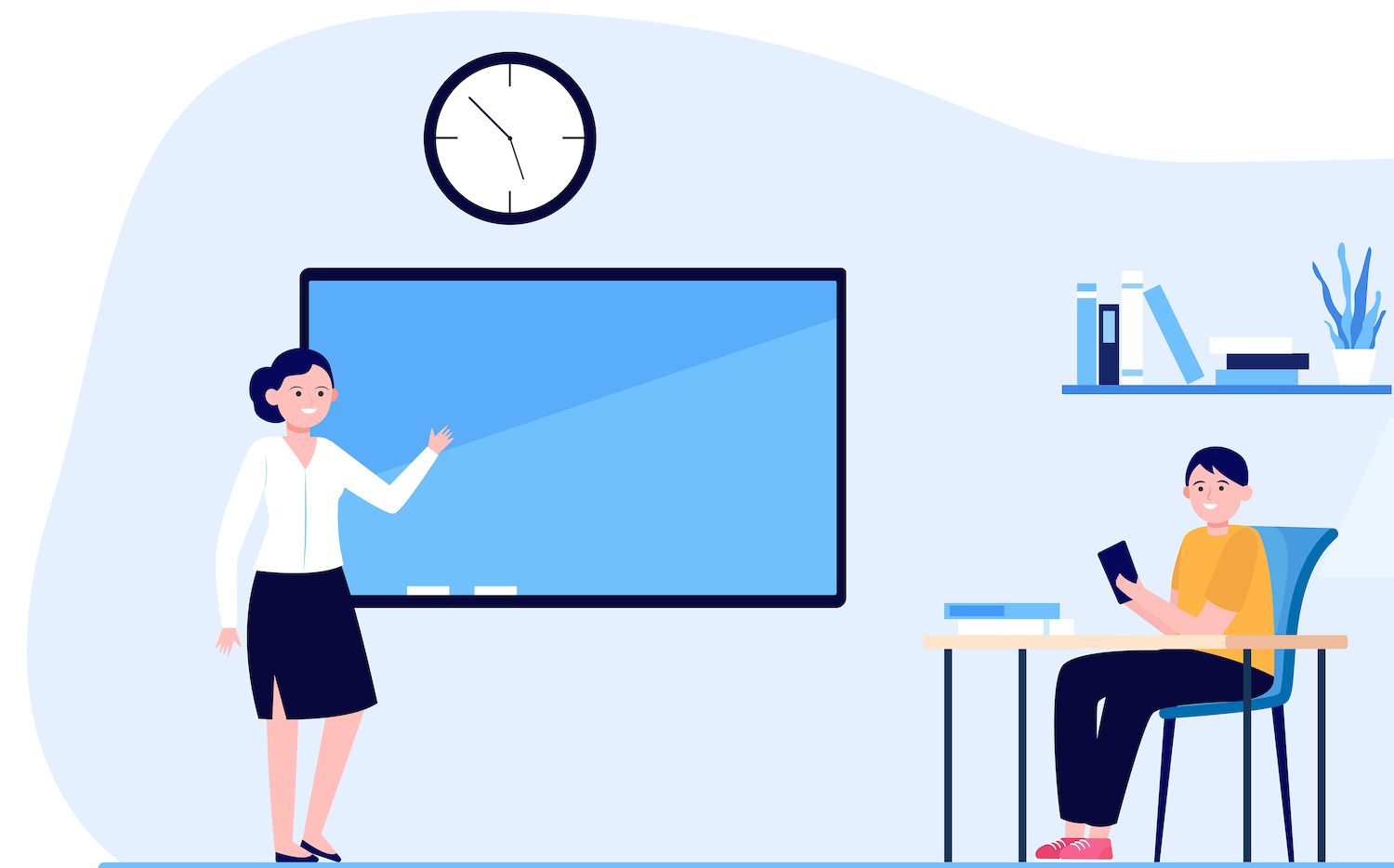
After you've clicked"Send" once you've clicked the "Send" button You will receive this details:
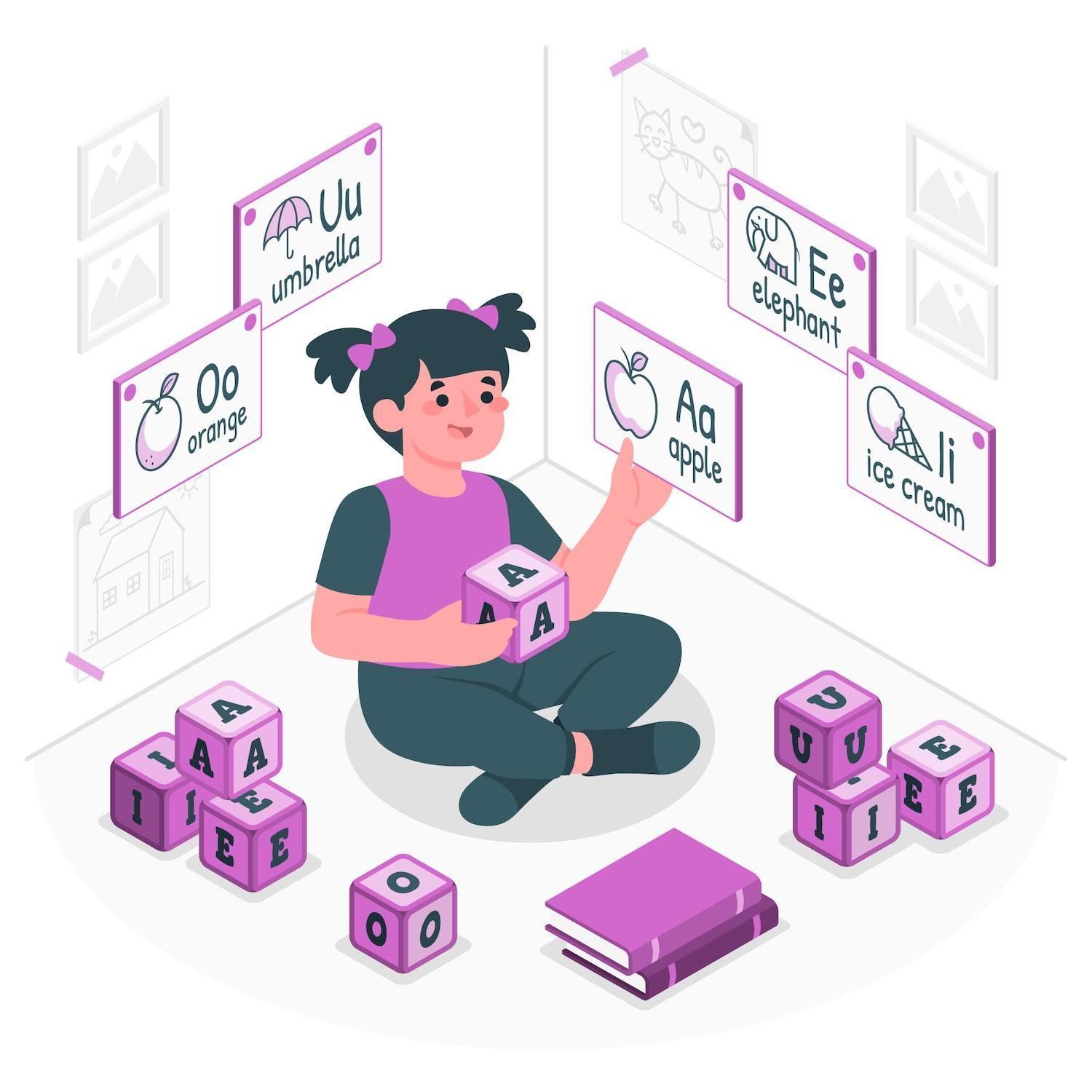
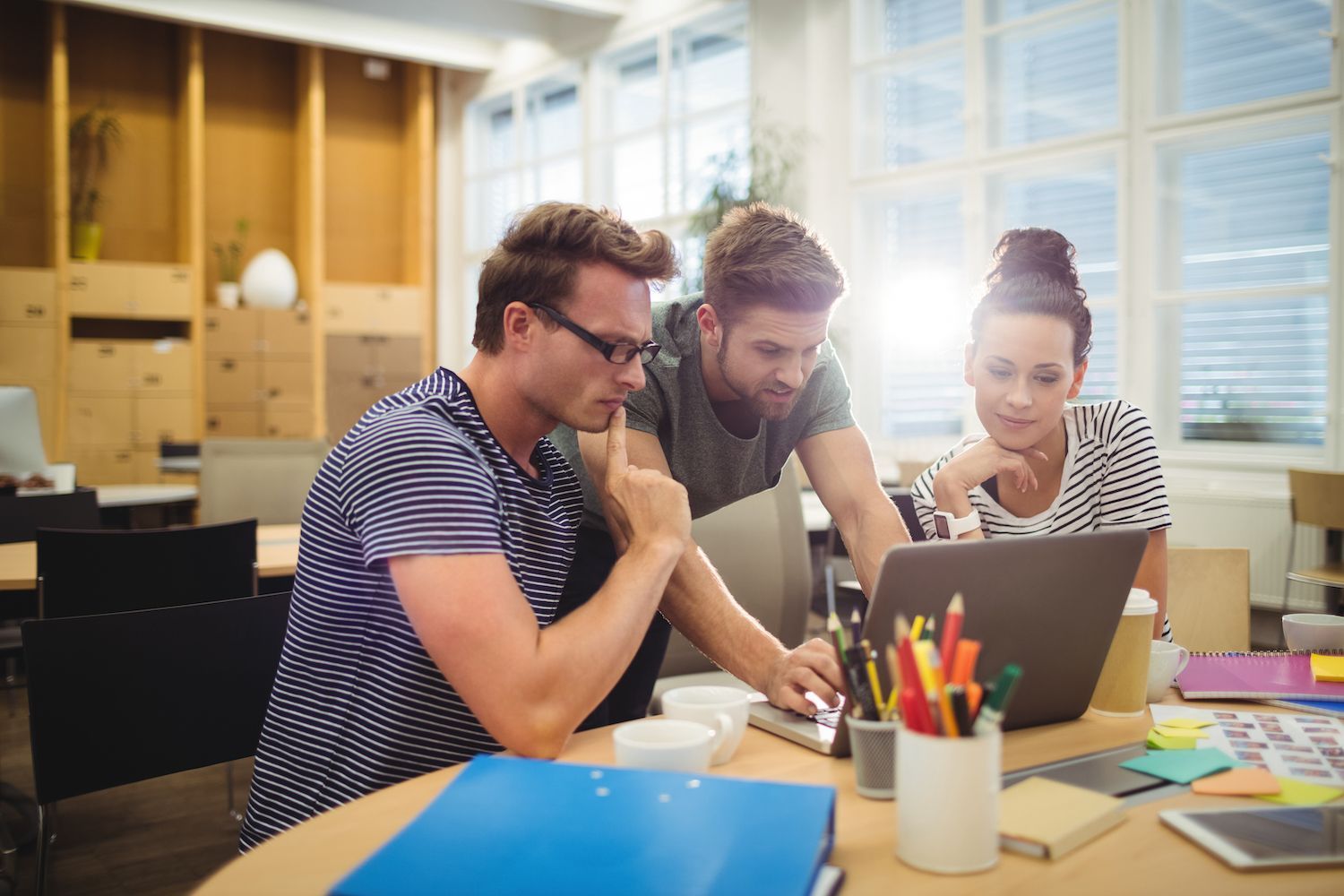
Which is the most efficient method of authenticating APIs using Sanctum?
Security of your API is dependent on authentication. your API. Laravel assists with this by providing the security feature included in the Sanctum token. It can serve as an intermediaryware. It protects APIs with tokens created when users log into their accounts by using the right login and username. Make sure that you are aware that the user will not be capable of accessing an API secured by token.
The first step for adding authentication is to add the Sanctum package by using the below code:
composer require laravel/sanctum
Put up the Sanctum configuration file:
php artisan vendor:publish --provider="Laravel\Sanctum\SanctumServiceProvider"
Then, you can add the token of Sanctum into middleware. Within in the app/Http/Kernel.php file, it is recommended to apply the following classes, and substitute middlewareGroups
with the following code to access the protected middleware group' API.
use Laravel\Sanctum\Http\Middleware\EnsureFrontendRequestsAreStateful;
protected $middlewareGroups = [ 'web' => [ \App\Http\Middleware\EncryptCookies::class, \Illuminate\Cookie\Middleware\AddQueuedCookiesToResponse::class, \Illuminate\Session\Middleware\StartSession::class, // \Illuminate\Session\Middleware\AuthenticateSession::class, \Illuminate\View\Middleware\ShareErrorsFromSession::class, \App\Http\Middleware\VerifyCsrfToken::class, \Illuminate\Routing\Middleware\SubstituteBindings::class, ], 'api' => [ EnsureFrontendRequestsAreStateful::class, 'throttle:api', \Illuminate\Routing\Middleware\SubstituteBindings::class, ], ];
The next step is to design UserController. UserController
and then add the code to generate the token for authentication.
php artisan make:controller UserController
After creating the UserController
, navigate to the app/Http/Controllers/UserController.php file and replace the existing code with the following code:
Prior to attempting to authenticate, it is necessary to establish an account on behalf of a particular user, using seeders. This command creates a users table document.
php artisan make:seeder UsersTableSeeder
Inside the database/seeders/UsersTableSeeder.php file, replace the existing code with the following code to seed the user: insert([ 'name' => 'John Doe', 'email' => '[email protected]', 'password' => Hash::make('password') ]);
Get started now by implementing this guideline:
php artisan db:seed --class=UsersTableSeeder
The final step to complete the authentication procedure is to install the middleware designed to secure the routing. Review the routes/api.php file, and then include the route for your product into it. routes/api.php file and include the route from your product in middleware.
use App\Http\Controllers\UserController; Route::group(['middleware' => 'auth:sanctum'], function () Route::apiResource('products', ProductController::class); ); Route::post("login",[UserController::class,'index']);
When you've made a fresh route by using the middleware it'll display an error from the server internals when you try to download the software.
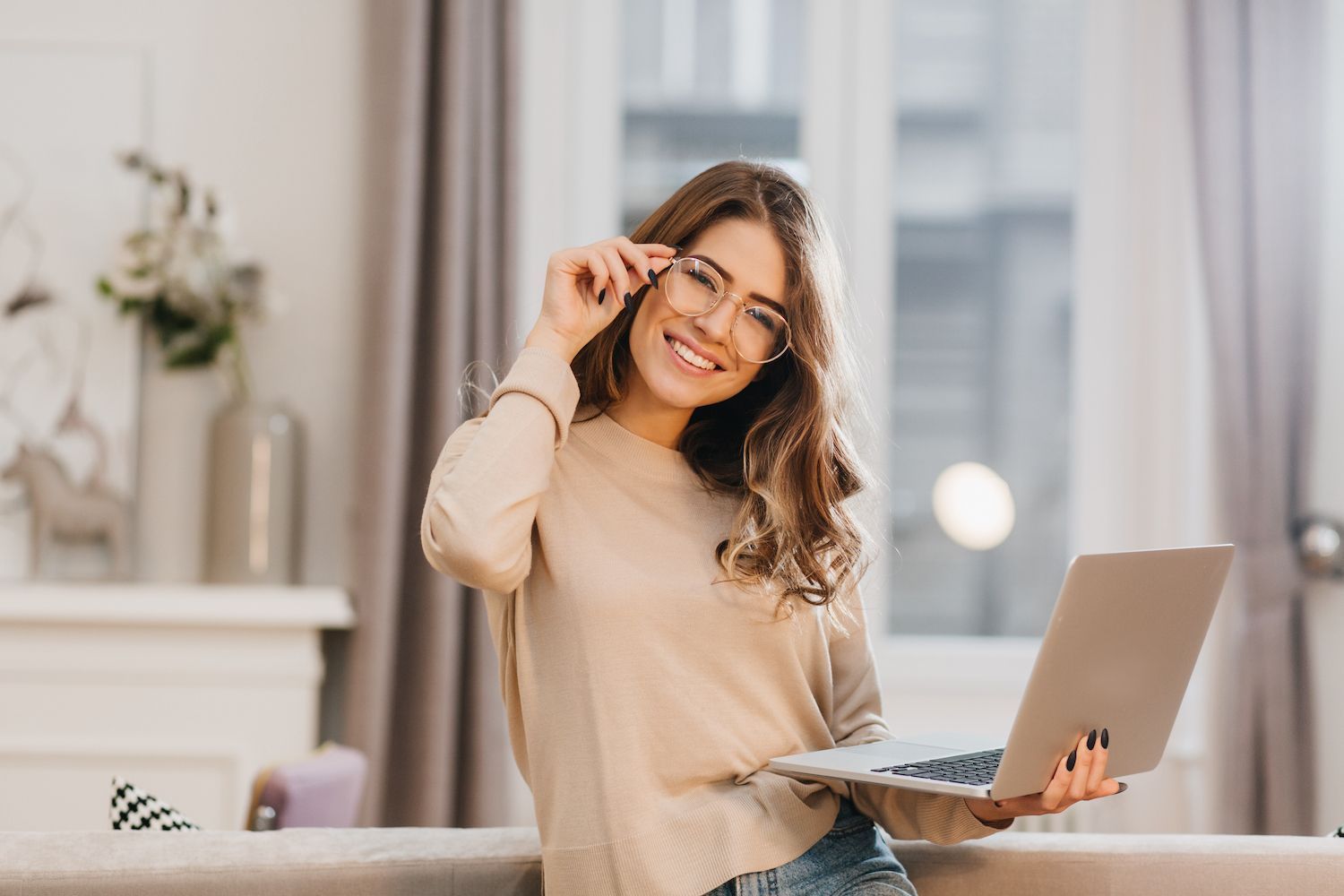
Once you've successfully logged in to your account, you'll get a personal token for use with your account. When you use it, make sure to include your HTTP header. It then checks your identity before working. You can send a POST request to http://127.0.0.1:8000/api/login with the following body:
"email":"[email protected]", "password":"password"
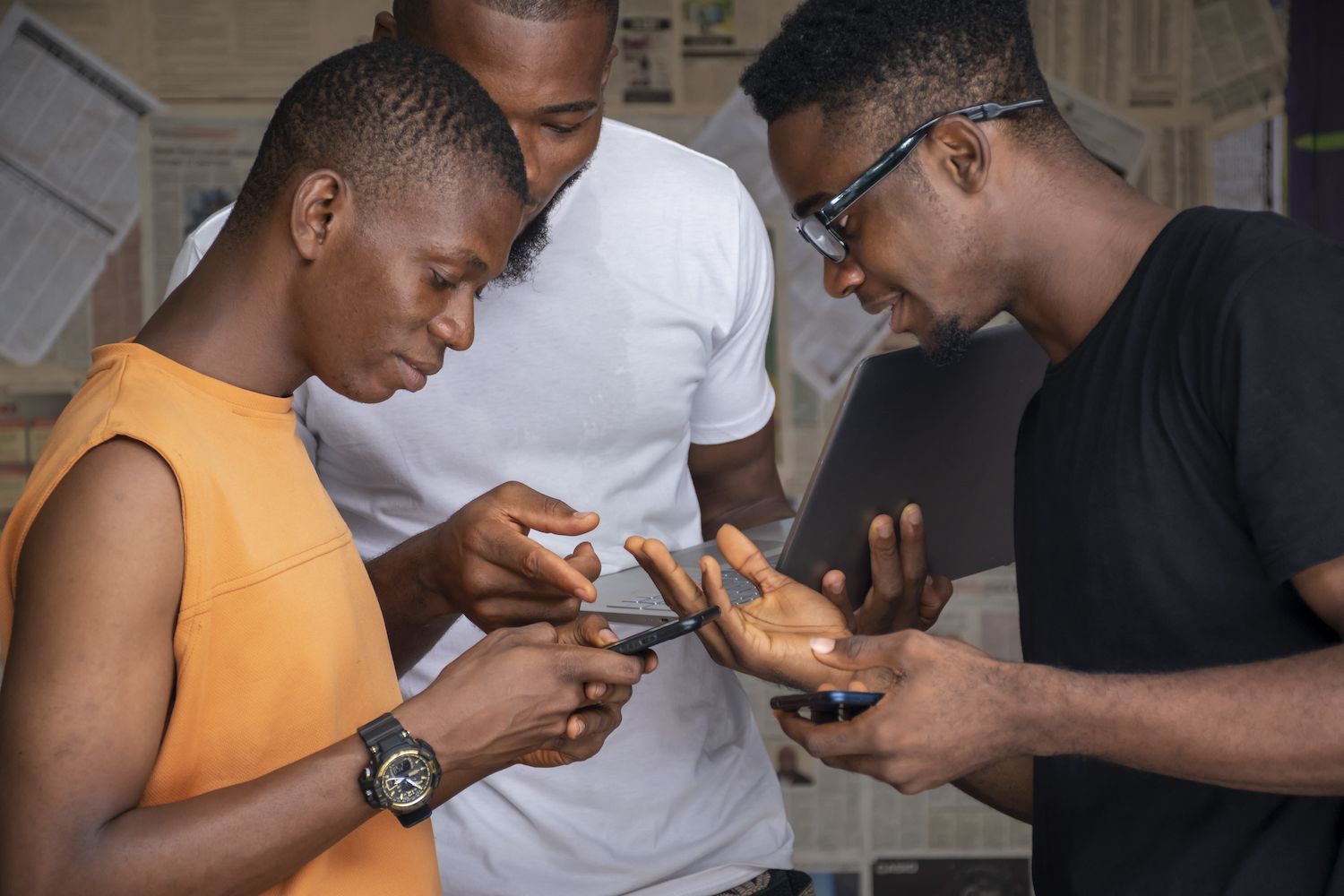
Utilize the token you got as a bearer token, and include it in the header for Authorization.
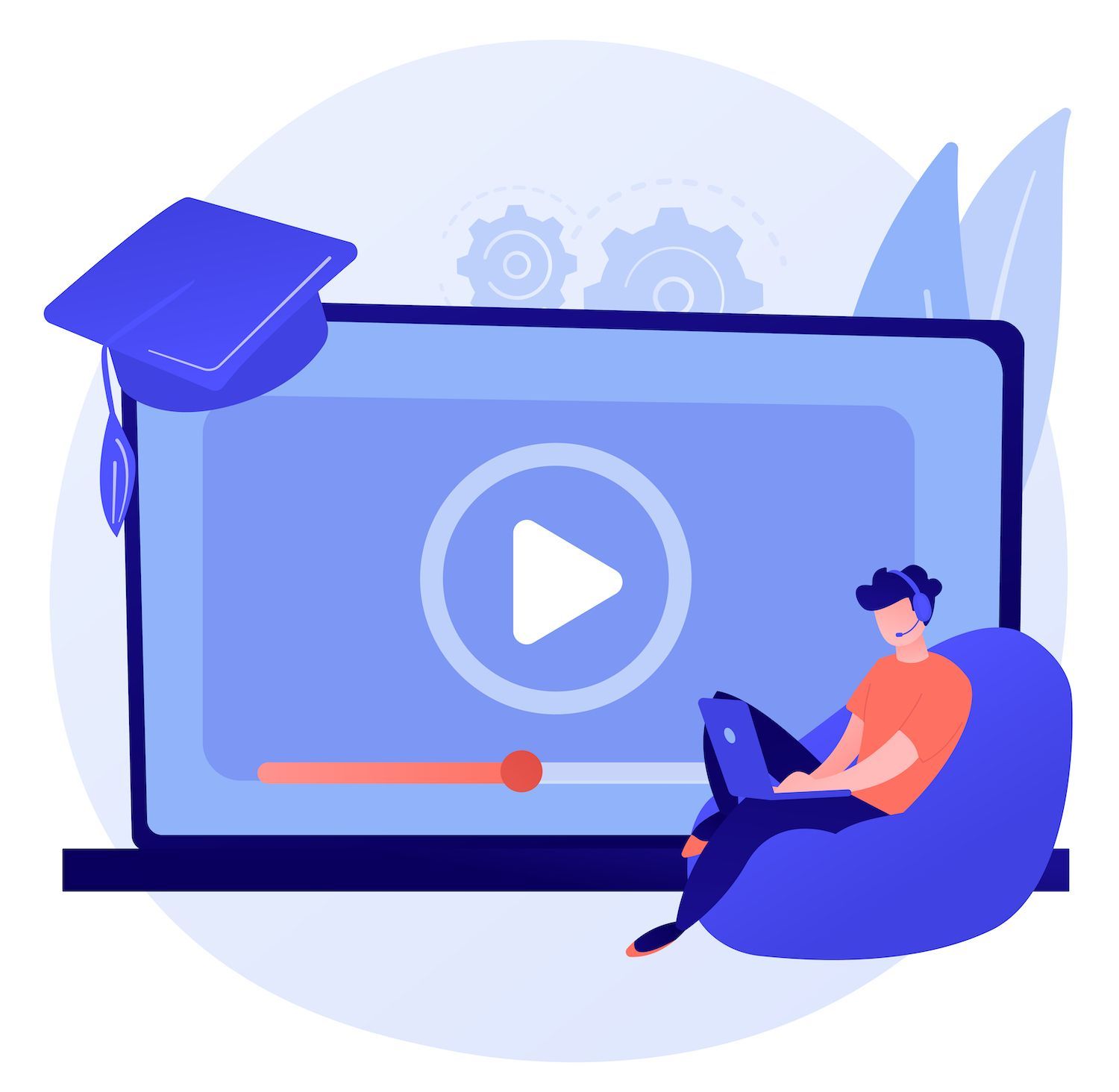
What are you able to do to correct API Errors
An error code by itself will not be enough. An error message that is readable by an individual is necessary. Laravel offers a variety of options to solve the issue. Utilize a fallback, catch block, or send a custom response. The following code you put into the UserController UserController
shows this.
if (!$user || !Hash::check($request->password, $user->password)) return response([ 'message' => ['These credentials do not match our records.'] ], 404);
Summary
The Laravel Eloquent Model makes it easy to develop and test the API and then test it. The mapping of objects offers the capability to connect easily with databases.
Furthermore, it works as a middleware. The token Sanctum created by Laravel helps protect APIs in a very short time.
- My dashboard is simple to control and configure inside My dashboard. My dashboard
- Support is available 24/7.
- The most reliable Google Cloud Platform hardware and network are powered by Kubernetes to increase the capacity
- High-end Cloudflare integration that speeds the process as well as increase security
- Global reach through greater than 35 data centers as with more than 275 points of contact across the world
The original article appeared on this website
The article was published on here
This post was first seen on here