Laravel API: Create and Test an API for Laravel -- (r)
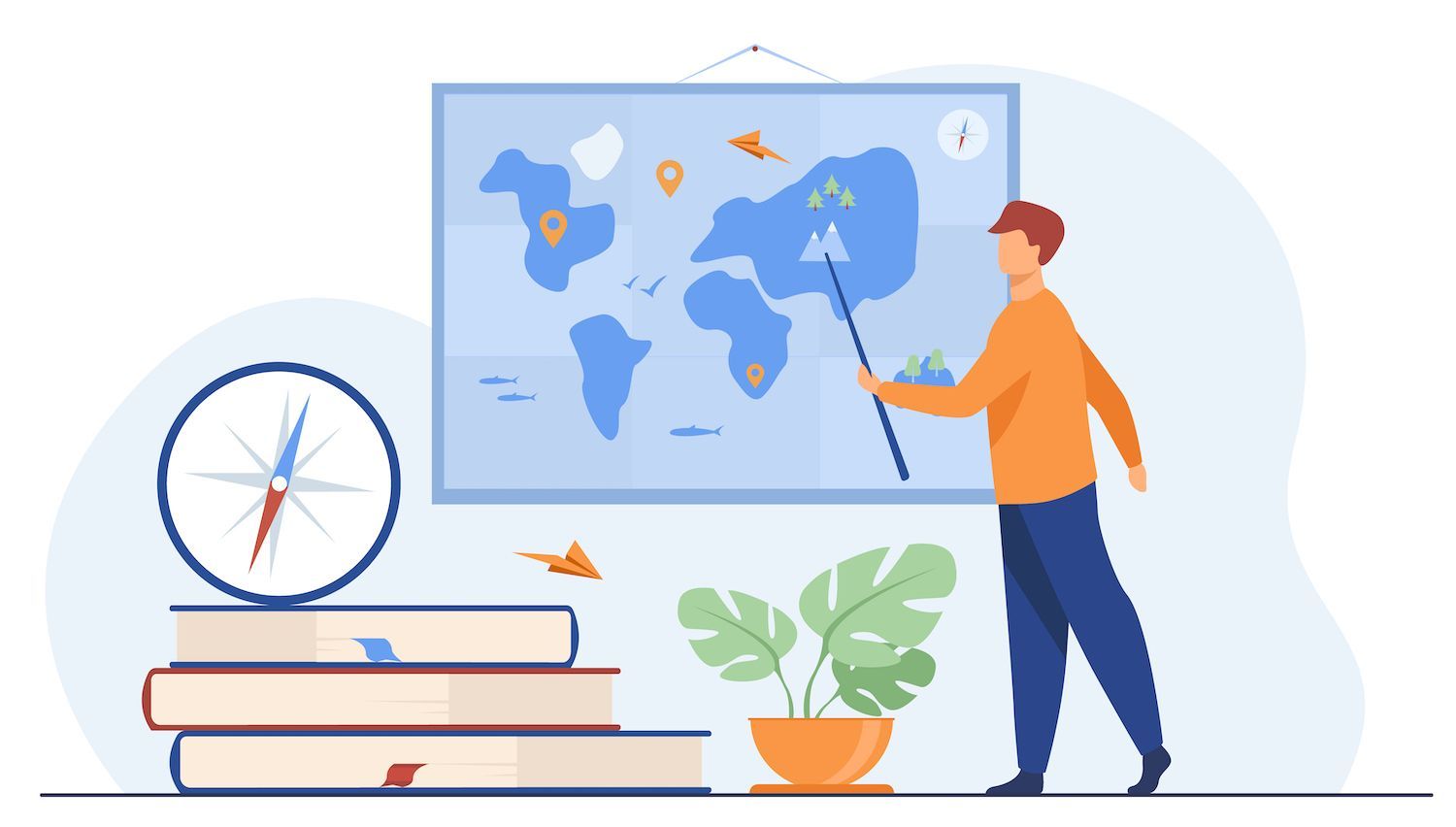
Send the news to
Laravel Eloquent is an easy approach to interface with your database. It's an object-relational mapping (ORM) which simplifies the complexity of databases through providing a framework that permits you to interact with tables.
In this way, Laravel Eloquent has excellent tools to create and test APIs, which can aid you to create APIs. In this hands-on article you'll learn how simple to create and test APIs in Laravel.
The prerequisites
To get going, here's what you'll need:
API Basics
Start by creating an entirely new Laravel project with the composer
:
composer create-project laravel/laravel laravel-api-create-test
To begin the server run the following command. This runs the application server at port 8000:
cd laravel-api-create-test php artisan serve
It is highly recommended to take a look at the screen below:
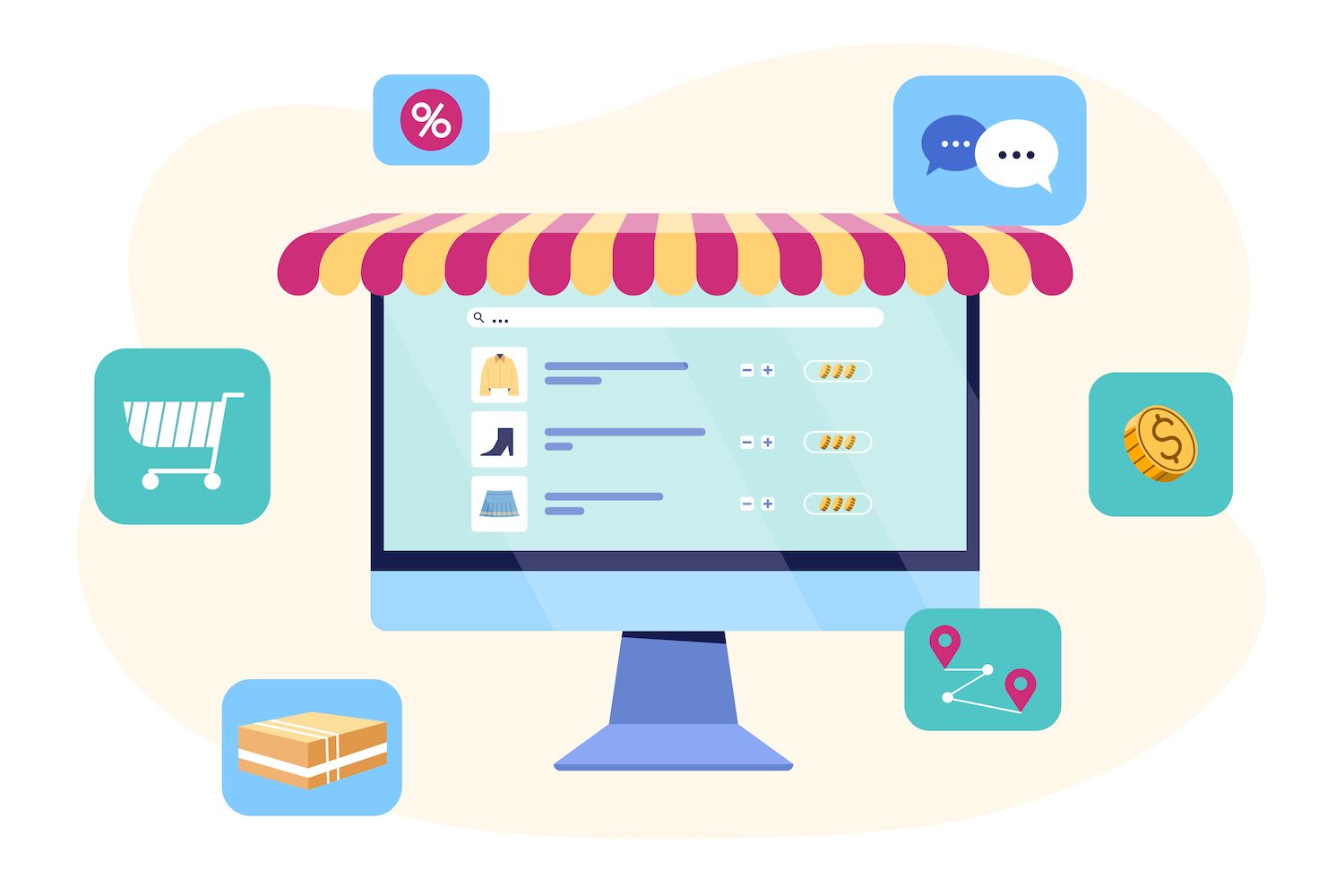
Then, create a model with a "-m
flag to permit the migration using the code in the following:
php artisan make:model Product -m
Then, upgrade your file in order to include the necessary field. Add title and description fields for the product model and these two table fields inside the database/migrations/date_stamp_create_products_table.php file.
$table->string('title'); $table->longText('description');
The next step is making these fields fully fillable. Inside app/Models/Product.php, make title
and description
fillable fields.
protected $fillable = ['title', 'description'];
How Do You Make the Controller
Create a controller file to control the product with the following command. This will create the app/Http/Controllers/Api/ProductController.php file.
php artisan make:controller Api\\ProductController --model=Product
Create the logic for creating and retrieving items. Within the index method index
method, you can include the following code in order to search for each item.
Product = $products = All :(); return the response ()->json(["status" is"false" and "products" is more than $products);
Then, you need to add an StoreProductRequest
class that will store the latest items within your database. The class that follows should be placed at the top of the same file.
public function store(StoreProductRequest $request) $product = Product::create($request->all()); return response()->json([ 'status' => true, 'message' => "Product Created successfully! ", 'product' => $product ], 200);
The next step is to start the process of creating the request. You can do by executing this commands:
php artisan make:request StoreProductRequest
If you want to add validations, you can use the app/Http/Requests/StoreProductRequest.php file. It is a demo in which there's no validation.
How Do You Create A Route
The final step before trying out the API is to create a new route. In order to do this, include this code into the routes/api.php file. Add to the file the usage
declaration in the top of the file and the the Route
assertion in the body:
use App\Http\Controllers\Api\ProductController; Route::apiResource('products', ProductController::class);
Before you begin testing the API, make sure that your Products table in your database. If not then create it with a control panel like XAMPP. It is also possible to run the following commands to migrate the database:
php artisan migrate
What is the best way to test an API
Before testing the API, ensure that the authorize
method inside the app/Http/Requests/StoreProductRequest.php is set to return true
.
Create a new product using Postman. Start by hitting a POST
request to this URL: http://127.0.0.1:8000/api/products/. Because this is an request to POST an
request for the creation of a brand new product, you need to provide an JSON object containing a name and description.
"title":"Apple", "description":"Best Apples of the world"
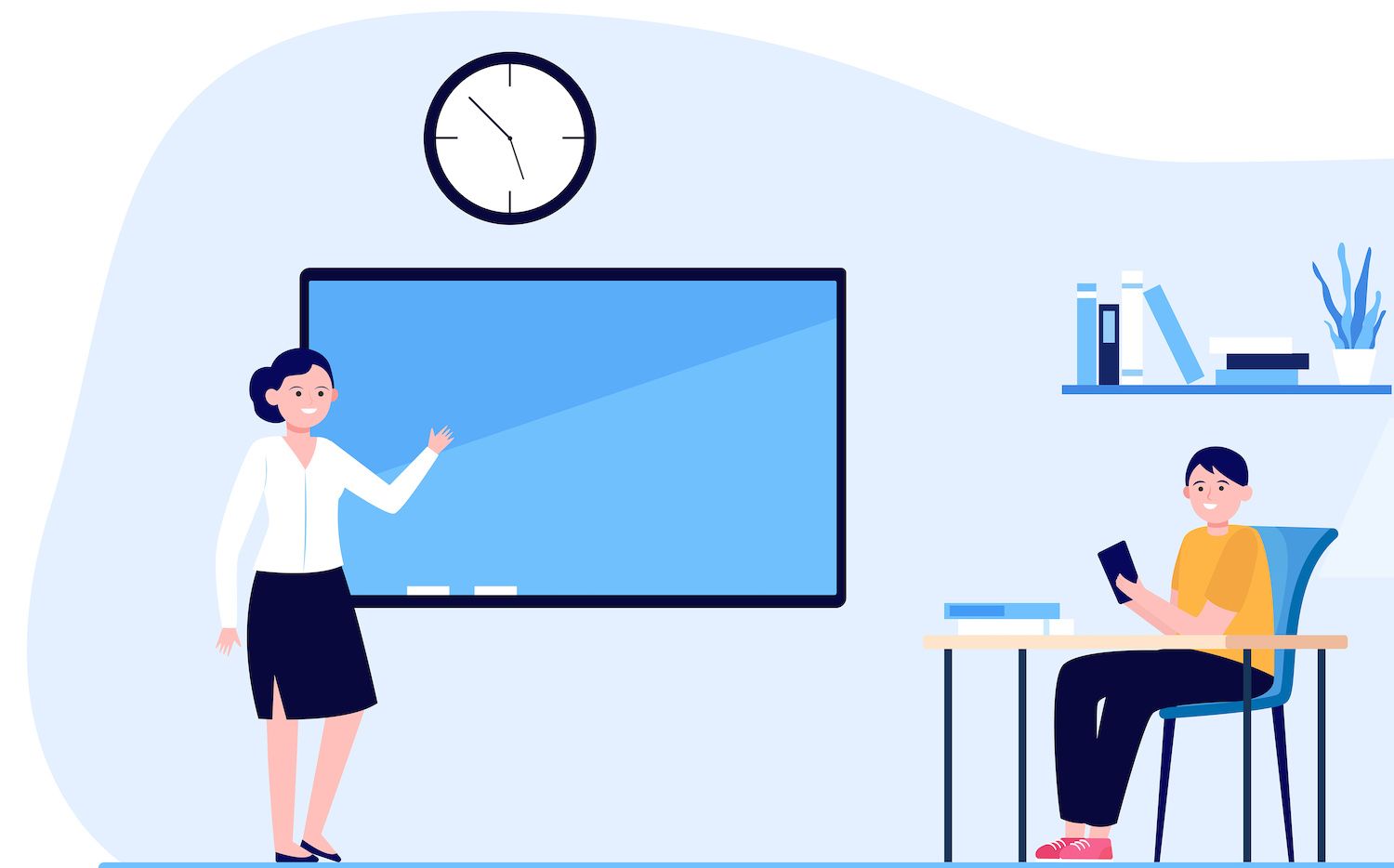
Once you have clicked"Send" after you click the "Send" button, you will get this information:
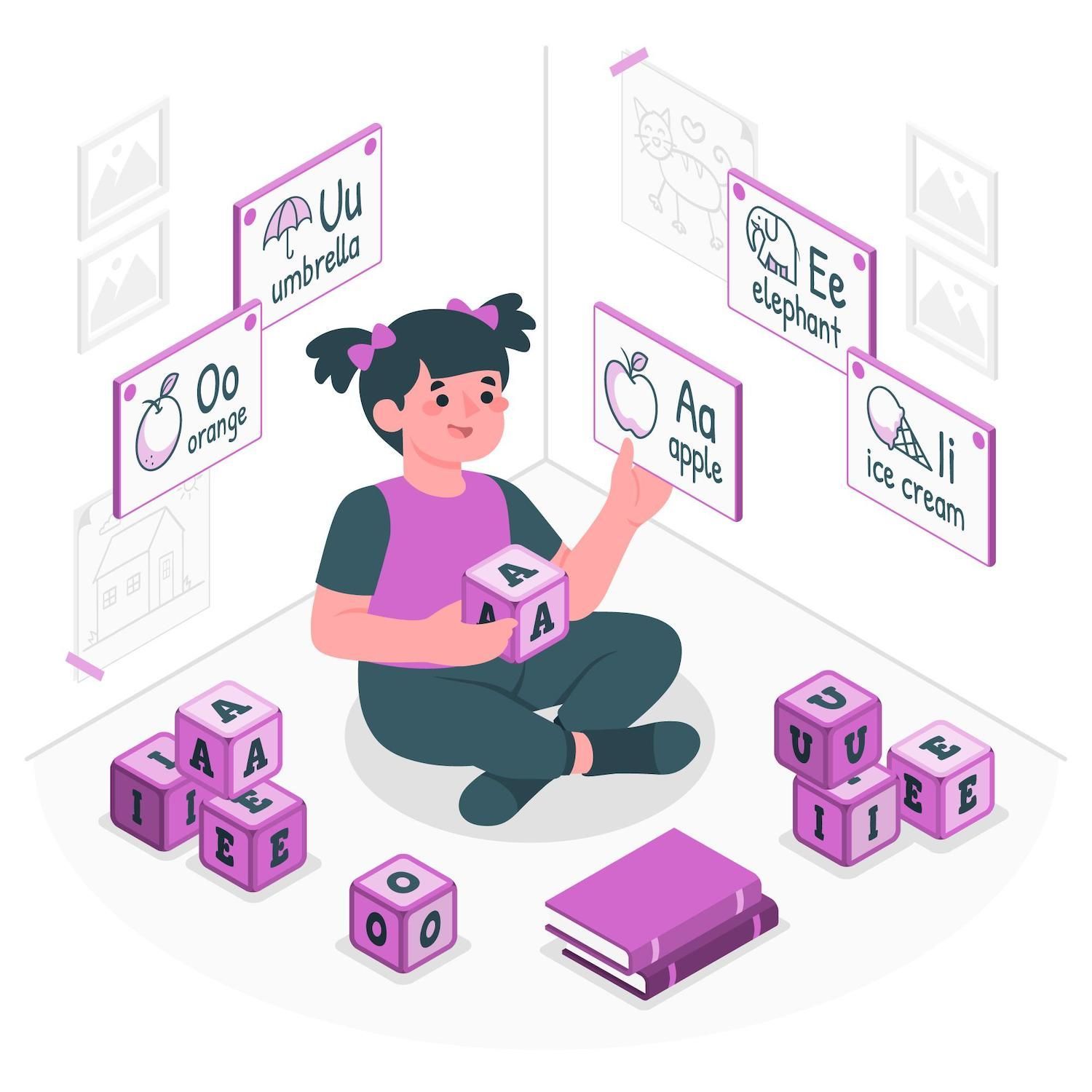
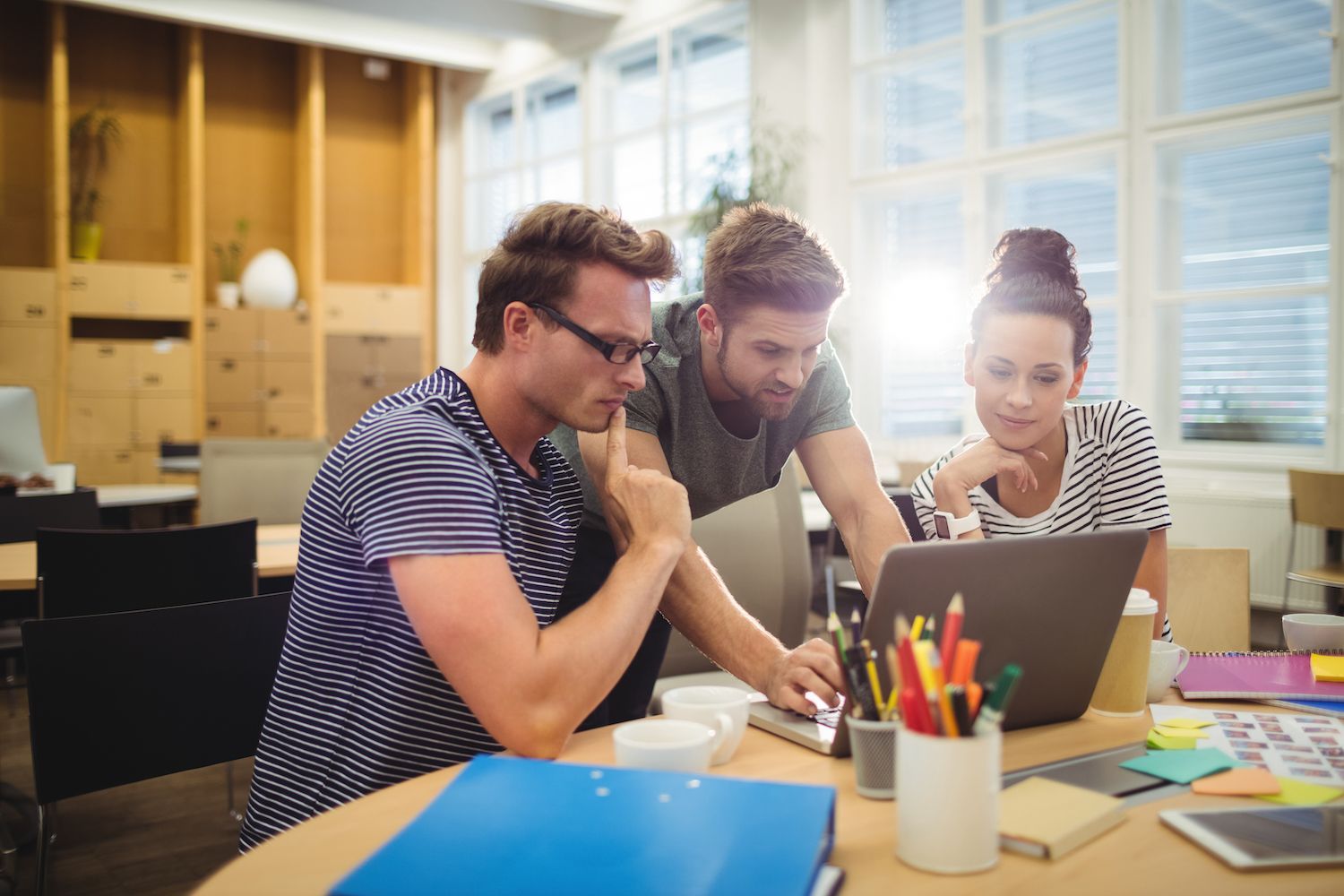
What's the most effective method of authenticating APIs using Sanctum?
Authentication is crucial when securing your API. Laravel facilitates it with the feature that is included in the Sanctum token, which can be used as an intermediateware. It safeguards APIs by using tokens created when users log in with the proper user name and password. Make sure that you are aware that the user is not able to access the secure API without the token.
The first step in adding authentication is to add the Sanctum package with the following code:
composer require laravel/sanctum
Then, make available the Sanctum configuration file:
php artisan vendor:publish --provider="Laravel\Sanctum\SanctumServiceProvider"
Following that, include Sanctum's token in middleware. In the app/Http/Kernel.php file, make use of the following class, and substitute middlewareGroups
with the code below for the protected middleware group' API.
use Laravel\Sanctum\Http\Middleware\EnsureFrontendRequestsAreStateful;
protected $middlewareGroups = [ 'web' => [ \App\Http\Middleware\EncryptCookies::class, \Illuminate\Cookie\Middleware\AddQueuedCookiesToResponse::class, \Illuminate\Session\Middleware\StartSession::class, // \Illuminate\Session\Middleware\AuthenticateSession::class, \Illuminate\View\Middleware\ShareErrorsFromSession::class, \App\Http\Middleware\VerifyCsrfToken::class, \Illuminate\Routing\Middleware\SubstituteBindings::class, ], 'api' => [ EnsureFrontendRequestsAreStateful::class, 'throttle:api', \Illuminate\Routing\Middleware\SubstituteBindings::class, ], ];
The next step is to build the UserController
and then add the code to generate the token needed to authenticate.
php artisan make:controller UserController
After creating the UserController
, navigate to the app/Http/Controllers/UserController.php file and replace the existing code with the following code:
Before you test the authentication process, you must create an account on behalf of a user by using seeders. This command will create a user table document.
php artisan make:seeder UsersTableSeeder
Inside the database/seeders/UsersTableSeeder.php file, replace the existing code with the following code to seed the user: insert([ 'name' => 'John Doe', 'email' => '[email protected]', 'password' => Hash::make('password') ]);
Start the Seeder right now by using this instruction:
php artisan db:seed --class=UsersTableSeeder
The final step to complete the authentication procedure is to utilize the middleware created to secure the routing. Explore routes/api.php file and add the product's route to it. routes/api.php file and include the product's route within the middleware.
use App\Http\Controllers\UserController; Route::group(['middleware' => 'auth:sanctum'], function () Route::apiResource('products', ProductController::class); ); Route::post("login",[UserController::class,'index']);
Once you've added a route using the middleware, you'll encounter an error from the server internals if you try to fetch products.
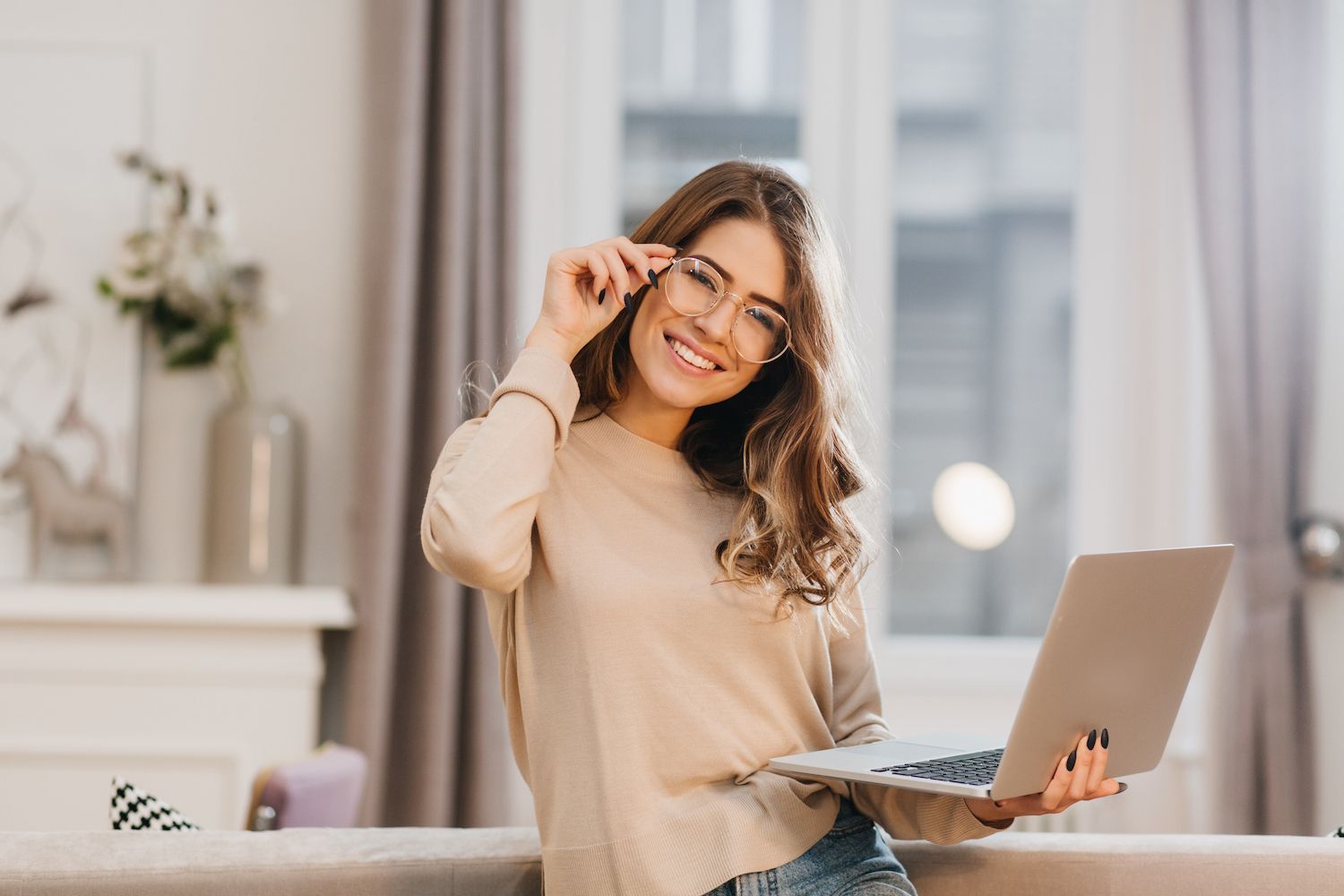
After you've logged into your account, you'll be provided with a token for your account. If you put it as an HTTP header. It will then verify your identity and start working. You can send a POST request to http://127.0.0.1:8000/api/login with the following body:
"email":"[email protected]", "password":"password"
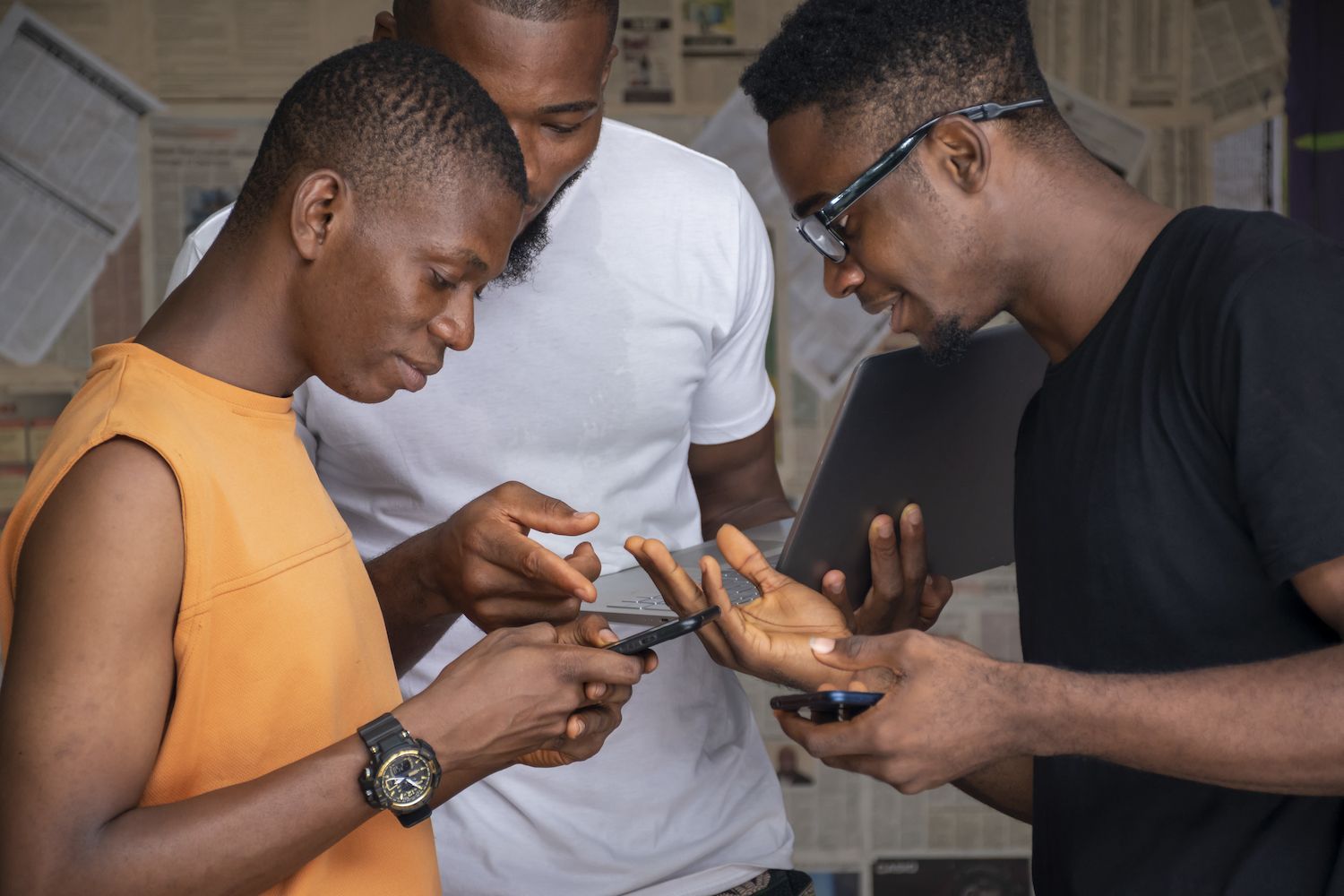
Make use of the token that you have obtained as a Bearer token after which you can add it to the Authorization header.
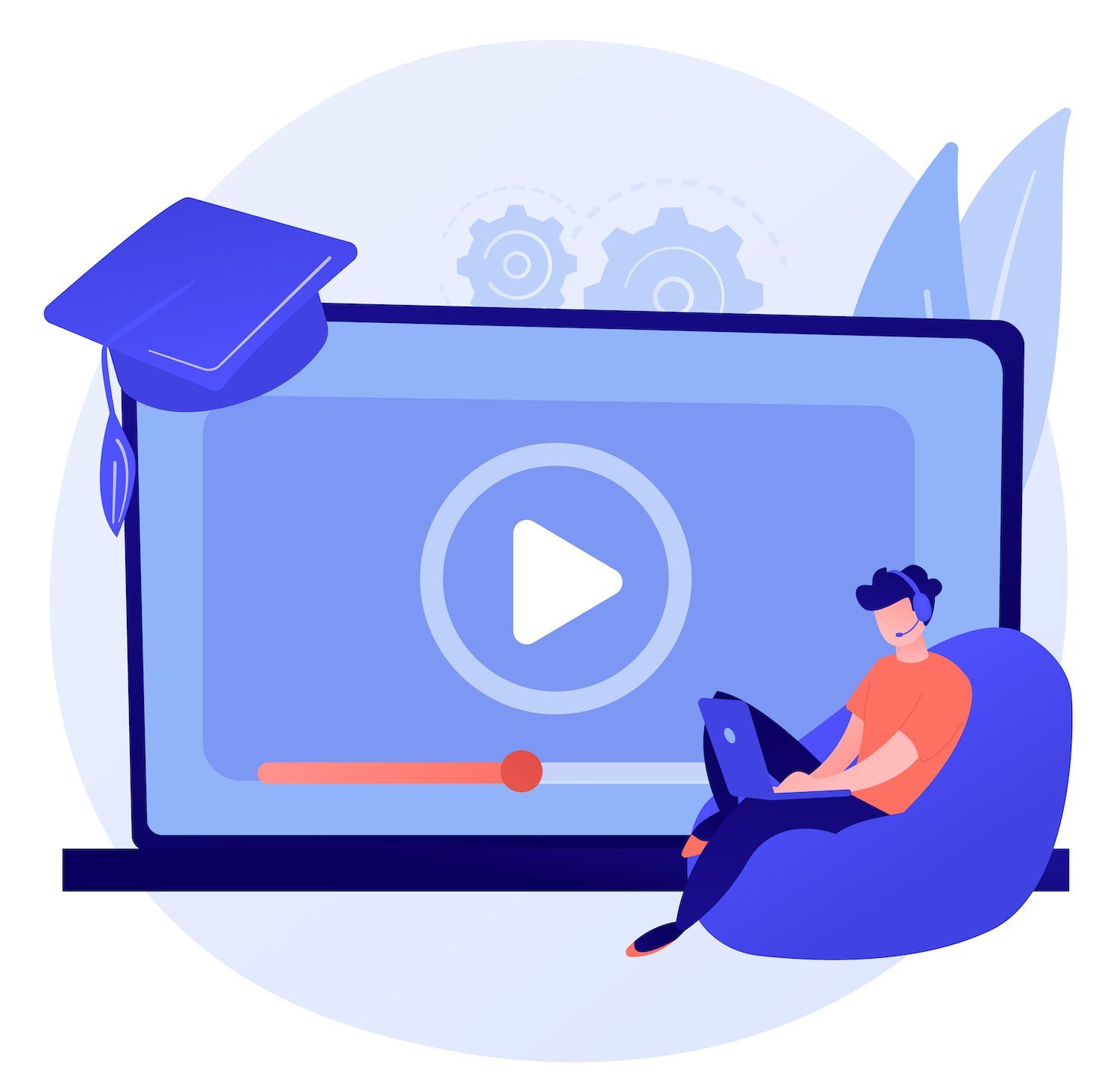
What to do about API Errors
An error code by itself will not be enough. An error message that is easily read by a human is required. Laravel offers a variety of solutions to the problem. Use a fallback, catch block or send a customized response. The following code you put into UserController UserController
illustrates this.
if (!$user || !Hash::check($request->password, $user->password)) return response([ 'message' => ['These credentials do not match our records.'] ], 404);
Summary
The Laravel Eloquent Model makes it easy to create, test the API, and then test it. Mapping objects gives the ability to easily interact with databases.
Furthermore, acting as middleware The token Sanctum from Laravel can help you protect your APIs in a short time.
- The My dashboard is easy to set up and manage in My dashboard. My dashboard
- 24/7 expert support
- The most efficient Google Cloud Platform hardware and network, driven by Kubernetes for maximum scalability
- High-end Cloudflare integration to speed up and improve security
- Global reach with more than 35 data centers and greater than 275 points of presence around the world
Article was first seen on here