Iterate like a Pro The complete how-to guide for Python Iterables (r)
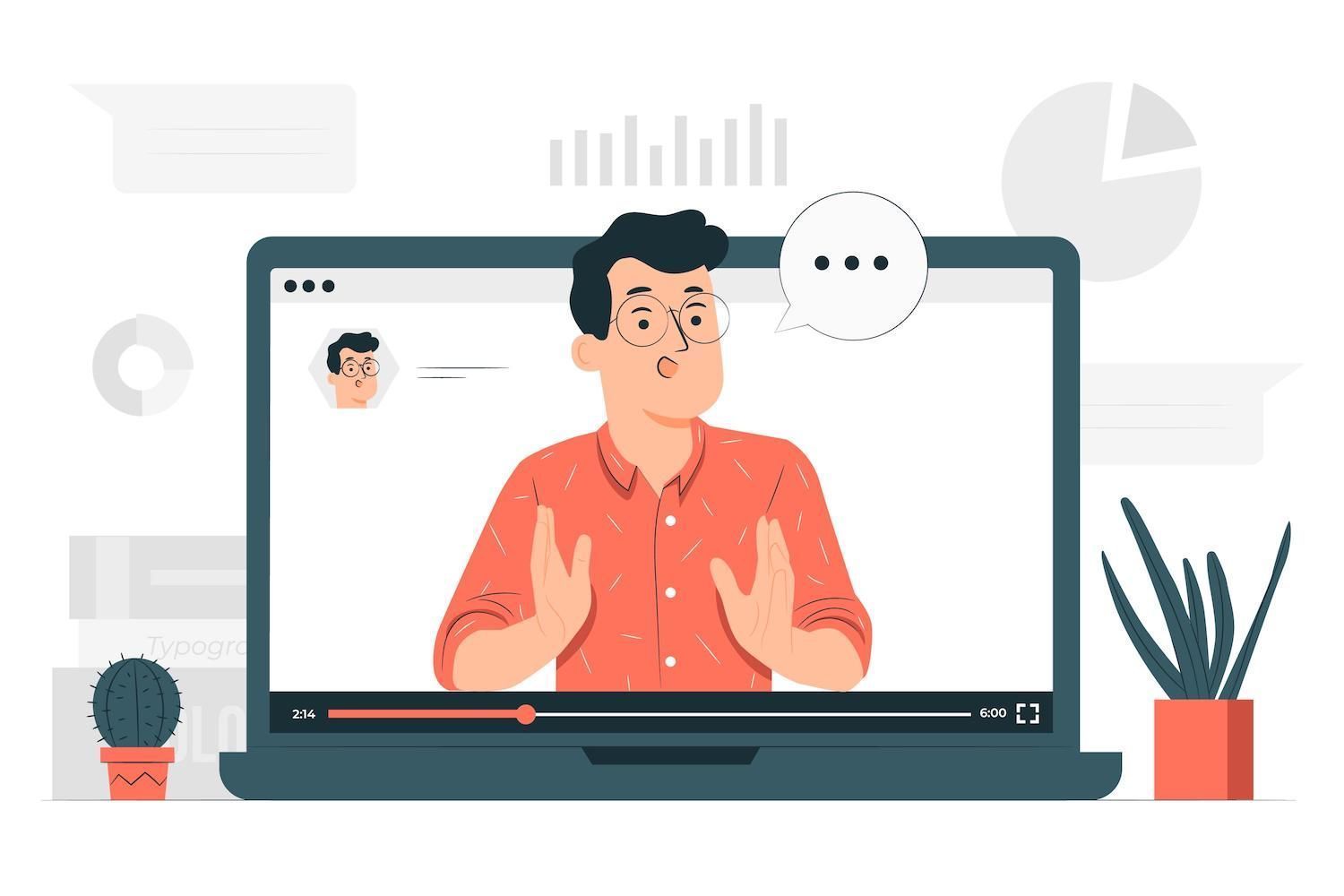
-sidebar-toc>
In the case of Python programming, figuring out the basic concepts of programming as well as how to use iterables efficiently is a crucial aspect that will help you to learn how to code effectively. Iterables are objects allow the user to repeat. They permit the continuous movements of components within the limits of their individual. This is why it's the ideal tool for getting access to and modifying components within data structures.
What is the best way to loop through Python Iterables
In Python is an programming language which allows users to run through a variety of iterative kinds using the for
loop. It allows you to navigate through the sequence of operations, but also specific elements in sets, lists, as well as Dictionarys.
1. An inventory of items that can be looped
Lists are organized collections of items that allow simple iteration with an for
loop.
fruits_list = ["Apple", "Mango", "Peach", "Orange", "Banana"] for fruit in fruits_list: print(fruit)
The code below, fruit
is an iterator that is employed to scan every element on the list, printing every one. It is ended with the assessment of the element that is last on the list. The code generates the following output:
Apple Mango Peach Orange Banana
2. Then iterating on iterating Tuple
Like lists, they're immutable. You can experiment using them in the same way as you do with lists.
fruits_tuple = ("Apple", "Mango", "Peach", "Orange", "Banana") for fruits in fruits_tupleprint(fruit)
In this instance this case, it is the loop that for
is looping across the tuple. Each time iteration, values of variables fruit
is changed to reflect the values of the current part of the tuple. The code outputs an output that looks like:
Apple Mango Peach Orange Banana
3. Looping through Sets
Sets are unsorted collection composed of various elements. Sets are traversable using the for
loop.
fruits_set = "Apple", "Mango", "Peach", "Orange", "Banana" for fruit in fruits_set: print(fruit)
In this example in this particular instance this particular instance, this is that loop for
loop which runs through the whole set. But, as the sets are not sorted, and therefore unordered and sorted, the order in which iterations occur might differ to the order that elements were assigned inside the set. Each time the variables are the fruit variables. Each iteration
will base their numbers on the elements currently present in the set. This code can produce results similar to one below (the quantity of elements might differ):
Mango Banana Peach Apple Orange
4. Repetition of Strings
Strings are the characters in the same story, and can be looped, each character being played at each time.
string = "" for string char print(char)print(char)
The code below iterates over"" within the text "" after which each character is displayed on one line. When the process is repeated, the variable character
will be changed in order to be a match for the character currently in the string. The program that generates this output:
KN s T
5. It is a Traversing Of the Dictionary of English
Utilizing the for loop and the for loop, the
loop can be used in conjunction with the for loop to construct lists, sets or tuples using strings. But, this differs when it comes to dictionaries as they utilize key-value pairs for storing items. They are a fantastic option for looping since they can be looped in a variety of different ways. The following are some methods you could use to explore a Python dictionary:
- The keys are altered:
countries_capital = "USA": "Washington D.C.", "Australia": "Canberra", "France": "Paris", "Egypt": "Cairo", "Japan": "Tokyo" for country in countries_capital.keys(): print(country)
This program generates a listing of countries_capital
which includes the names of countries where they serve as the keys, while their respective capitals constitute the main components. The loop is a for
loop scans through the keys in the countries_capital
dictionary using the key() technique. keys()
method. The result of this method is called a view, which shows the keys that are contained in the dictionary. It is easy to browse through all keys. In the event that you use the same loop the key variable country
will be changed to the values of the current key. The program will generate the outputs listed below.
USA Australia France Egypt JapanJapan
- The process of transferring value
countries_capital = "USA": "Washington D.C.", "Australia": "Canberra", "France": "Paris", "Egypt": "Cairo", "Japan": "Tokyo" for capital in countries_capital.values(): print(capital)
In the code above, which can be seen in the above code, the code for
it loops through the values in the countries_capital
dictionary using the numerals(). it is the value()
method. This method produces an object with a view of a listing of the values contained in the dictionaries. It allows you to browse through all possible options. Every time that you repeat, the capital variable capital
returns to the most current number on the list. The code you use will produce the following output:
Washington D.C. Canberra Paris Cairo TokyoTokyo
- The procedure of creating key-value pairs
countries_capital = "USA": "Washington D.C.", "Australia": "Canberra", "France": "Paris", "Egypt": "Cairo", "Japan": "Tokyo" for country, capital in countries_capital.items(): print(country, ":", capital)
The above code shows how to loop through the value and key of the countries_capital
dictionary by using the items()
method. The method returns an item view.()
method returns an item view that displays a list of keys and value tuples that are present in the dictionary. Within the for
loop, each iteration is capable of decoding a key/value pairing that's currently in the list. The variables capital and country
capital and capital
share the same key and value and key, respectively. This code is expected to produce output like this:
USA : Washington D.C. Australia : Canberra The City of CanberraFrance : Paris Egypt : Cairo Japan : Tokyo
Advanced Iteration with Enumerate() Python Python
Another method to iterate Python iterables and return the index and the value of the elements by using iterate(). iterate()
function. Look at the code below.
fruits_list = ["Apple", "Mango", "Peach", "Orange", "Banana"] for index, fruit in enumerate(fruits_list): print(fruit, index)
The result is:
Apple 0 Mango 1 Peach 2 Orange 3 Banana 4
Its Function Enumerate
function allows you to define an index which will serve as the starting place, and is a bit more than zero
in the course of the iteration. It is possible to modify the sample as follows:
fruits_list = ["Apple", "Mango", "Peach", "Orange", "Banana"] for index, fruit in enumerate(fruits_list, start = 2): print(fruit, index)
This output can be defined by:
Apple 2 Mango 3 Peach 4 Orange 5 Banana 6
This illustration shows the index that starts at the beginning used in this process, enumerate
utilizes zero-based indexing for the iterable similar to native lists do. It simply places the index that is at the start of the list on the very first item in the list, and continues through to the final.
How do I find the best way to setup Python Generators
Generators were specifically designed to work with Python iterables that allow users to construct generators, without having to explicitly create models. They are constructed in, like lists sets along with dictionary sets. Generators are able to generate value as they progress, using the logic of generators.
Generators use a yield
statement to return created value at the rate of. Here's a diagram of an iterable generator
def even_generator(n) Counter = 0 while counter is n when counter %2 == 0:yield counter counter= 1for num in even_generator(20):print(num)
The code described defines an even-generator
function which generates even numbers which range from the number 0
up to a particular number
through yield. yield
expression. The loop creates the values runs the same process again by using this numbers in the
iterator in order to guarantee the evaluation of all values within the specified zone. The program creates an odd-numbered table that spans from and 20.
up to 20
that is based on:
0 2 4 6 8 10 12 14 16 18 20
The efficiency can be increased through the use of generator expressions. For instance it is possible to design an engine which can include loop logic
cube (num * 3 for number within the range(1 6)) to print C within cube (print(c) in cubeprint(c)
This is where you can define this cube as an variable cube
for the purpose of displaying the results of a computer program that calculates the worth of the cube in the range 1 and 6. It then loops through the range of values and releases the outcomes of the calculation each prior to the next. The result will be in the form of:
1 8 27 64 125
How do you build custom iterables?
Python lets you further modify the iterative process through the use of iterators. Iterator objects are an element of the iterator protocols. There are two options: __iter__()
and the following()
. Iterator method is referred to as the iter()
method returns an object that is an iterator, whereas the method known as"__next()
returns the next value for the container, which can be iterable. Here's an excellent illustration of an iterator created using Python:
even_list = [2, 4, 6, 8, 10] my_iterator = iter(even_list) print(next(my_iterator)) # Prints 2 print(next(my_iterator)) # Prints 4 print(next(my_iterator)) # Prints 6
If you're using the method of iter() method, you will use an iter()
method to create an iterator ( my_iterator
) within the following list. To gain access to every item in the list, you will create an iterator object with the following()
method. Because lists are collections that are organized they return all elements in a sequential order.
Iterators with a customized design can prove useful when you want to manage large files that you can't transfer into memory simultaneously. Because memory is costly and has restrictions in space as well as space restrictions. it is possible to employ an iterator to manage all the components of data on its own and not load all the data into memory.
Multi Functions
Python utilizes functions for moving through the components in an array. List functions that are common used comprise:
sum
is the amount of an iterable. As the case is it's comprised of numbers (integers floating numbers points, integers, as well as complex numbers)All
returnstrue
every time an element from the list is authentic. If all the elements listed in the list are authentic elements returnthe result True. False.
.the entire
isvalid
for elements that can be iterated have valid. If there isn't then it isthe value of.
.max
provides the highest value that is calculated with an iterable arraymin
-- The minimum amount to be used in an an irrational collection.len
Retrieves the complete length of an iterableappend
adds additional value to the very top on the listing.
This image showcases the capability with this checklist
even_list = [2, 4, 6, 8, 10] print(sum(even_list)) print(any(even_list)) print(max(even_list)) print(min(even_list)) even_list.append(14) # Add 14 to the end of the list print(even_list) even_list.insert(0, 0) # Insert 0 and specified index [0] print(even_list) print(all(even_list)) # Return true only if ALL elements in the list are true print(len(even_list)) # Print the size of the list
It's the result.
30 True10 2[2, 4 6, 8, 10, 14] [0 2, 4 7 8 14] False7
In the earlier example, Append function will be used to append. This function can be utilized to join 14 numbers ( 14) into the middle of table. Insert function lets you define an index that you wish to insert. function Insert
function lets you define the index you want to insert. So, even_list.insert(0 (0, (0,)
inserts zero
to index [0insert[0](0 0, 0)
. The statement print(all(even_list))
returns false
because there is a 0
value in the list, interpreted as false
. Finally, print(len(even_list))
outputs the length of the iterable.
Advanced Iterable Operations
Python has advanced capabilities that make for a more simple process. Here are some of the features.
1. List Comprehensions
List comprehension can be used to build list comprehensions simply by including actions for each item on the list. This is an example
my_numbers = [11, 12, 13, 14, 15, 16, 17, 18, 19, 20] even_number_list = [num for num in my_numbers if num%2 == 0] print(even_number_list)
This code snippet demonstrates how an array of numbers known by the name my_numbers
is generated using numbers ranging between 11
up to twenty
. The reason for this list is to make a totally different list. even_number_list
with only even integers. To achieve this, make use of the list comprehension that returns the number of integers that are represented from my_numbers
only when the number is even. This clause
part of the logic to return the even numbers.
It is a result that:
[12, 14, 16, 18, 20]
2. Zip
Python zip() function Python zip()
function combines several iterables to produce Tuples. Tuples have the capacity to keep a wide range of variables, and can be altered for a long time. Find out how to mix iterables using Zip()
:
fruits = ["apple", "orange", "banana"] rating = [1, 2, 3] fruits_rating = zip(rating, fruits) print(list(fruits_rating))
In this instance, fruits_rating
pairs each fruit's rating giving one iterable. The output of the code will be:
[(1, 'apple'), (2, 'orange'), (3, 'banana')]
The code acts as an instrument for evaluating diverse fruit. The first list ( fruits
) depicting the fruits, while another listing provides ratings scales between one and three.
3. Filter
Another function which is superior to the filter function, which is also referred to as one that is dependent on two arguments that consist of functions and an iterable. The function is applied to all elements of the iterable. This creates an iterable that only contains the elements where it gives an genuine
outcome. This is an example of the method of filtering an array of numbers within the defined range and return only those which are:
def is_even(n): return n%2 == 0 nums_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] even_list = filter(is_even, nums_list) print(list(even_list))
Similar to what you've read in the earlier code, it starts by constructing an algorithm, is_even
which computes an even number, which is passed back to it. Then, you create an integer listing that's between 1 and 10-and the list of nums
. At the end of the day the list is created, and you make a fresh list called an the even_list
that uses its filter()
function to implement the algorithm you defined in the prior list in order to display only those portions of the list that match. The result is this:
[2, 4, 6, 8, 10]
4. Map
Similar to filters()
, Python's map()
function takes an iterable and an argument. The latter is in the form of arguments. In lieu of returning the whole array of elements in the previous iterable, it generates an entirely new one that results from the procedure that was applied to elements of the prior one. If you're looking to construct an uniqrupled list of numbers make use of using the maps()
function:
my_list = [2, 4, 6, 8, 10] square_numbers = map(lambda x: x ** 2, my_list) print(list(square_numbers))
In this instance, x
is the iterator which traverses the list, before changing it by using the square. Map() functions mapping()
function executes the process with the list's initial argument before running it with the help of mapping. Its output will be as follows:
[4, 16, 36, 64, 100]
5. Sorted
It is the sort
function that arranges the components of an iterable in a way which is specified (ascending or decreasing) and displays the result in an array. It takes into account three maximum parametersthe of which are iterable
, reverse
(optional) in addition to iterable reverse (optional) and iterable key
(optional). This is the reason reverse
will default to False
when you alter the value to the time of change.
and the components are listed as ascending order they appear. key
is a function which decides on a value to determine the order within which an element is placed. The element's value can be iterative and will always default to the number none.
.
Here's an example on how to apply the sort
function for different iterables:
# set py_set = 'e', 'a', 'u', 'o', 'i' print(sorted(py_set, reverse=True)) # dictionary py_dict = 'e': 1, 'a': 2, 'u': 3, 'o': 4, 'i': 5 print(sorted(py_dict, reverse=True)) # frozen set frozen_set = frozenset(('e', 'a', 'u', 'o', 'i')) print(sorted(frozen_set, reverse=True)) # string string = "" print(sorted(string)) # list py_list = ['a', 'e', 'i', 'o', 'u'] print(py_list)
The output is:
['u', 'o', 'i', 'e', 'a'] ['u', 'o', 'i', 'e', 'a'] ['u', 'o', 'i', 'e', 'a'] ['a', 'i', 'k', 'n', 's', 't'] ['a', 'e', 'i', 'o', 'u']
What can you do to address errors and Edge Cases Iterables
Edge cases are often encountered when programming in a variety of situations and you are advised to be prepared for them whenever you come across iterables. We'll look at a few possibilities you might come across.
1. Incomplete Iterables
In some cases, you may come across an empty iterable however, the logic of the program tries to handle the issue. This is a problem that can be solved by programming in order to eliminate errors. This is a good example of the use of a When-not
expression to verify that an item is not empty.
fruits_list = If the list does not appear to be fruit-related The list is not,print("The list isn't complete")
The result is.
The table isn't empty
2. Nested Iterables
Python can also create nested Iterables with iterable objects with additional iterables in their. For example, you can build lists of food items which contain various types of foodstuffs that are placed in a container like meats, vegetables and cereals. Let's look at the way to model the scenario by employing nesting iterables
food_list is [["kale", "broccoli", "ginger"], ["beef", "chicken", "tuna"], ["barley", "oats", "corn"]] to create an inner list of food items. Provide a list of things that can be included within the list:print(food)
In the above code you can see that, in the code below you can see that the food_list
variable is made up of three nested lists each one representing different food groups. The loop that iterates ( for inner_list in food_list
) is executed over the primary list, within the loop ( for food in the list at the bottom:
) iterates through every nested list and prints the food items in each list. The results of the program can be explained in the following way:
Kale broccoligingerchicken tunabarley oats,corn
3. Exception Handling
In Python iterables they provide the ability to handle exceptions. In this instance, you could look through an array of objects and spot an index defect
. It means that you're creating something that's out from the boundaries of what's possible. What should you do if you encounter this type of error? Utilize an attempt-to-exclude
block?
fruits_list = ["apple", "mango", "orange", "pear"] try: print(fruits_list[5]) except IndexError: print("The index specified is out of range. ")
The following code shows iterables of fruits are lists of fruit
iterables are made up of five sections with indices of five
to five
on the list. The expression you wish to verify test
is the one that contains the print
function. It tries to display the value on five of the iterable. five
is in the iterable, however, it doesn't exist. The exception clause runs and returns an error message. The console displays the error message:
The search result you're searching for is not within the range.
Summary
Learning how to iterate using Python is crucial for writing proficient and simple-to-read programming. Knowing the different ways to construct different kinds data structures by with generators, comprehensions, using built-in functions, makes you a proficient Python programmer.
Have we missed anything from the book? Please let us know by commenting in the section that follows!
Jeremy Holcombe
The Content and Marketing Editor for WordPress Web Developer and the Content writer. Aside from everything WordPress I like playing golf and on the beach or watching movies. Additionally, I'm tall. Problems ;).
The original post appeared on this site
The post was published on this site.
This post was first seen on here